
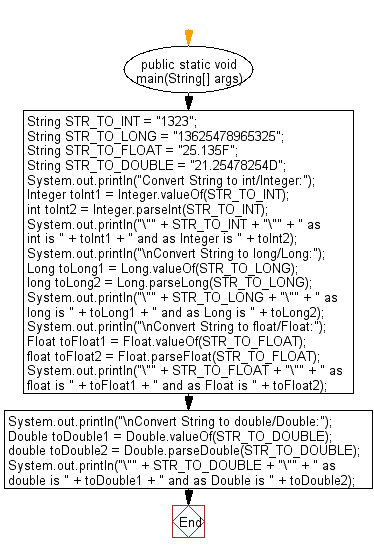
- Convert string to long integer in java algorithm update#
- Convert string to long integer in java algorithm code#
- Convert string to long integer in java algorithm free#
It is also efficient because valueOf() method implement Flyweight pattern (see Design Patterns courses) and maintains a pool of frequently used Long values, particularly in the range of -128 to 127, as shown below. you can pass an int to method expects long int i // Concatenate i with an empty string conversion is handled for you. Since Long.valueOf() does accept a long primitive, passing an int value to it is no problem at all, here you go :
Convert string to long integer in java algorithm free#
If you use Long.valueOf() then you don't need to explicitly cast an int to long, Why? because you can pass an int value to a method which is accepting primitive long.ītw, if you are not familiar with such fundamental rules of Java programming, I suggest taking these free Java online training courses from Udemy and Pluralsight. One of the better ways to convert an int primitive to the Long object is by using the valueOf() method of the Long class. In our case, it is still ok because the loop is running only 10 times, but if have to do this conversion say a million times, this approach will cause a problem, as explained by Joshua Bloch in his classic book, Effective Java 3rd Edition.

Convert string to long integer in java algorithm code#
So, if you want to use autoboxing, make sure you cast the int value to long first, don't worry though, if you forget compiler will ensure this :)Īnother thing to notice in the above code is that we are using auto-boxing in the loop, which can cause performance issue because a lot of Long objects will be created and discarded. String strTest 100 Try to perform some arithmetic operation like divide by 4 This immediately shows you a compilation error.
Convert string to long integer in java algorithm update#
You cannot pass an int to them because auto-boxing will not be able to convert an int to Long (see The Complete Java Masterclass - Update for Java 11), but, if you cast an int to long, then auto-boxing will take care of converting it to Long object, as shown below : There are two ways to convert String to Integer in Java, String to Integer using Integer.parseInt () String to Integer using Integer.valueOf () Let’s say you have a string strTest that contains a numeric value. Same is true for a method which is expecting a Long object. Long one = 10 // Type mismatch : cannot convert from int to Long In order to leverage autoboxing, you must typecast an int variable to long, otherwise, the compiler will complain about mismatch type, as shown below : 1 Note that using BigInteger as suggested below will remove the ability to use operators (+, -,, etc). For example, the static factory methodīoolean.valueOf(String) is almost always preferable to theĬonstructor Boolean(String).1. You can often avoid creating unnecessary objects by using staticįactory methods (Item 1) in preference to constructors on immutableĬlasses that provide both. since most of them reuse instances whenever it is possible making them potentially more efficient in term of memory footprint than the corresponding parse methods or constructors.Įxcerpt from Effective Java Item 1 written by Joshua Bloch: Generally speaking, it is a good practice to use the static factory method valueOf(str) of a wrapper class like Integer, Boolean, Long. Please note that the previous code can still throw a NumberFormatException if the provided String doesn't match with a signed long. Thanks to auto-unboxing allowing to convert a wrapper class's instance into its corresponding primitive type, the code would then be: long val = Long.valueOf(str) Similarly, we can convert the string to any other primitive data type: parseLong(): parses the string to Long parseDouble(): parses the string to double If we want to convert the string to an integer without using parseInt(), we can use valueOf() method. To cache values within a particular range. Note that unlike theĬorresponding method in the Integer class, this method is not required Likely to yield significantly better space and time performance byĬaching frequently requested values.
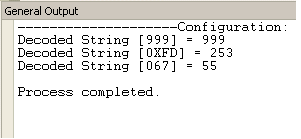
Used in preference to the constructor Long(long), as this method is New Long instance is not required, this method should generally be Returns a Long instance representing the specified long value. The best approach is Long.valueOf(str) as it relies on Long.valueOf(long) which uses an internal cache making it more efficient since it will reuse if needed the cached instances of Long going from -128 to 127 included.
